Constructing Other States using "0" State on Quantum Computing
As you may know, the interface of IBM's Quantum Composer or Qiskit/QASM only lets us to "reset" a quantum bit to $|0\rangle$ state. However, it's a must to have use other states when designing a quantum circuit. In this blog post, I'll introduce you how to create those and their state vectors.
Please study the sphere below. It is called Bloch Sphere and lets us imagine how does quantum gates changes a state into another one. On z-axis, we have two classical states: $0$, $1$. On y-axis, we have two complex states: $i$, $-i$. At last, on x-axis, we have two sign states: $+$, $-$.
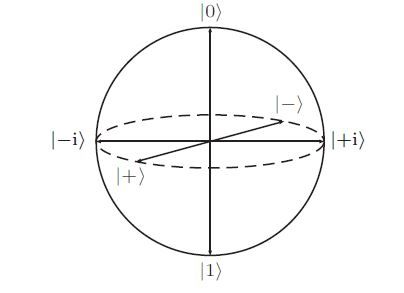
Classical States
In quantum world, $|0\rangle$ and $|1\rangle$ are known as classical states. Since resetting operation directly creates a $|0\rangle$ state, we will be only investigating on creating $|1\rangle$ state.
To have $|1\rangle$, we should turn our $|0\rangle$ vector by $\pi$ radians either on x-axis. So, we'll use an X gate to convert it.
from qiskit import QuantumRegister, QuantumCircuit
from numpy import pi
qbit = QuantumRegister(1, 'q')
circuit = QuantumCircuit(qbit)
circuit.reset(qbit[0])
circuit.x(qbit[0])
OPENQASM 2.0;
include "qelib1.inc";
qreg q[1];
reset q[0];
x q[0];
Sign States
With using Hadamard gate, we can construct a superposition between $|0\rangle$ and $|1\rangle$ which also equivalent to $|+\rangle$ or $|-\rangle$ states.
To construct a $|+\rangle$, let us reset a qubit to $0$ and then apply a Hadamard gate to it.
from qiskit import QuantumRegister, QuantumCircuit
from numpy import pi
qbit = QuantumRegister(1, 'q')
circuit = QuantumCircuit(qbit)
circuit.reset(qbit[0])
circuit.h(qbit[0])
OPENQASM 2.0;
include "qelib1.inc";
qreg q[1];
reset q[0];
h q[0];
To construct a $|-\rangle$, we need to input a $|1\rangle$ into Hadamard gate. Since we've already learned how to create each classic gate, let's implement it, and apply a H gate afterwards.
from qiskit import QuantumRegister, QuantumCircuit
from numpy import pi
qbit = QuantumRegister(1, 'q')
circuit = QuantumCircuit(qbit)
circuit.reset(qbit[0])
circuit.x(qbit[0])
circuit.h(qbit[0])
OPENQASM 2.0;
include "qelib1.inc";
qreg q[1];
reset q[0];
x q[0];
h q[0];
Complex States
Complex states are the edges of Bloch Sphere on y-axis. To create a complex state, we may firstly create a sign state (as we previously learned) and then apply an S gate which rotates $\pi/2$ radians on x-y plane. Outputs of S gate are $|i\rangle$, $|-i\rangle$ when $|+\rangle$, $|-\rangle$ given, respectively.
Let's construct a $|+\rangle$ state, and apply S gate into it to have $|i\rangle$ state.
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit
from numpy import pi
qbit = QuantumRegister(1, 'q')
circuit = QuantumCircuit(qbit)
circuit.reset(qbit[0])
circuit.h(qbit[0])
circuit.s(qbit[0])
OPENQASM 2.0;
include "qelib1.inc";
qreg q[1];
reset q[0];
h q[0];
s q[0];
Now, it is the turn to construct a $|-i\rangle$ state.
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit
from numpy import pi
qreg_q = QuantumRegister(1, 'q')
circuit = QuantumCircuit(qreg_q)
circuit.reset(qreg_q[0])
circuit.x(qreg_q[0])
circuit.h(qreg_q[0])
circuit.s(qreg_q[0])
OPENQASM 2.0;
include "qelib1.inc";
qreg q[1];
reset q[0];
x q[0];
h q[0];
s q[0];
As you see, we've constructed all the Quantum states using the single-input gates. I hope that helps!